Logging in and out
You can also log in and out with both Pay and PoS users using the SDK.
1. Logging in
Logging in works similarly to registration, you need to create a login flow and decorate it with user data, but luckily the SDK can fully hide all overhead from the client side this time.
let request = LoginRequest(identifier: phoneNumber, password: password)
vipasoPay.login(request: request) { result in
switch result {
case .success:
// Success: nothing to be returned, the SDK listener will trigger a new authentication state change event
case .failure(let error):
// Error: any server or network error
}
}
val request = LoginRequest(phoneNumber, password)
vipasoPay.login(request)
If you debug your networking calls, you should see the following traffic:
- HTTP request and response for retrieving the flow id in the background:
GET /a/self-service/login/api HTTP/1.1
HTTP/1.1 200 OK
Content-Type: application/json; charset=utf-8
{
"id": "b1f0d684-7c85-4efa-b8a6-6041e1e77eaa",
...
}
- HTTP request and response for logging in:
POST /a/self-service/login?flow=b1f0d684-7c85-4efa-b8a6-6041e1e77eaa HTTP/1.1
Content-Type: application/json
{
"identifier": "+439999999999",
"method": "password",
"password": "111111"
}
HTTP/1.1 200 OK
Content-Type: application/json; charset=utf-8
{
"session_token": "ory_st_ZXMeo8204gD0u3PbQu0DgsG3hBASzz8E",
"session": {
"id": "a29924fd-14d7-46ee-b329-73854e876405",
"identity": {
"id": "9a8cbfb3-87bd-4087-b48d-2569b1b43386",
...
},
...
},
...
}
Example screenshots from our development application:
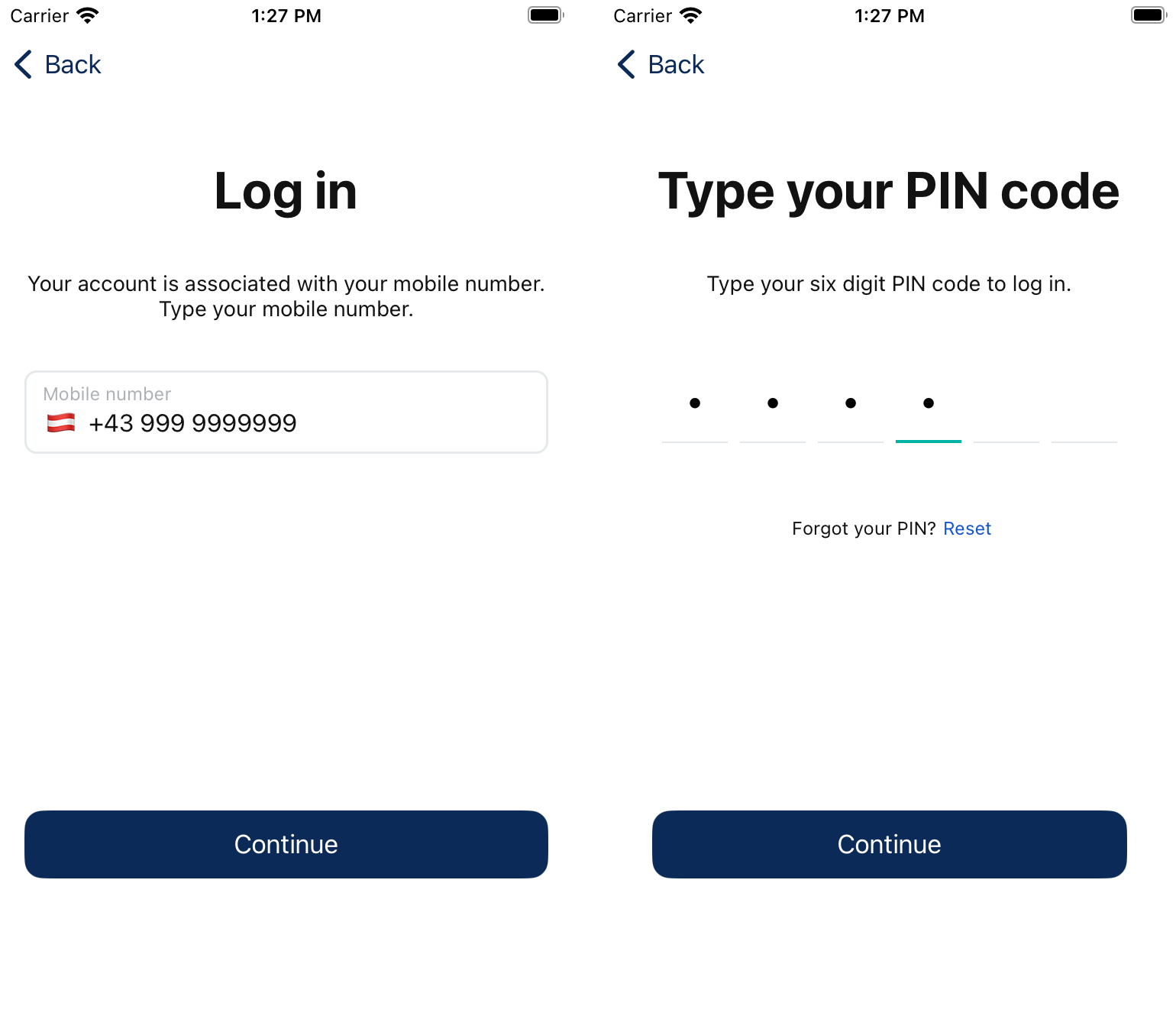
Note: In the end the SDK will automatically generate and save a local key pair and send the public key to the backend. This needs to happen for the user to be considered logged in. You will see a PUT
request to /identity/pki/client/certificates
at the end of the flow.
2. Logging out
Logout is synchronous and there is no backend communication involved. The SDK will clean up everything from its database and the keychain that are connected to the user.
vipasoPay.logout()
vipasoPay.logout()
Example screenshots from our development application:
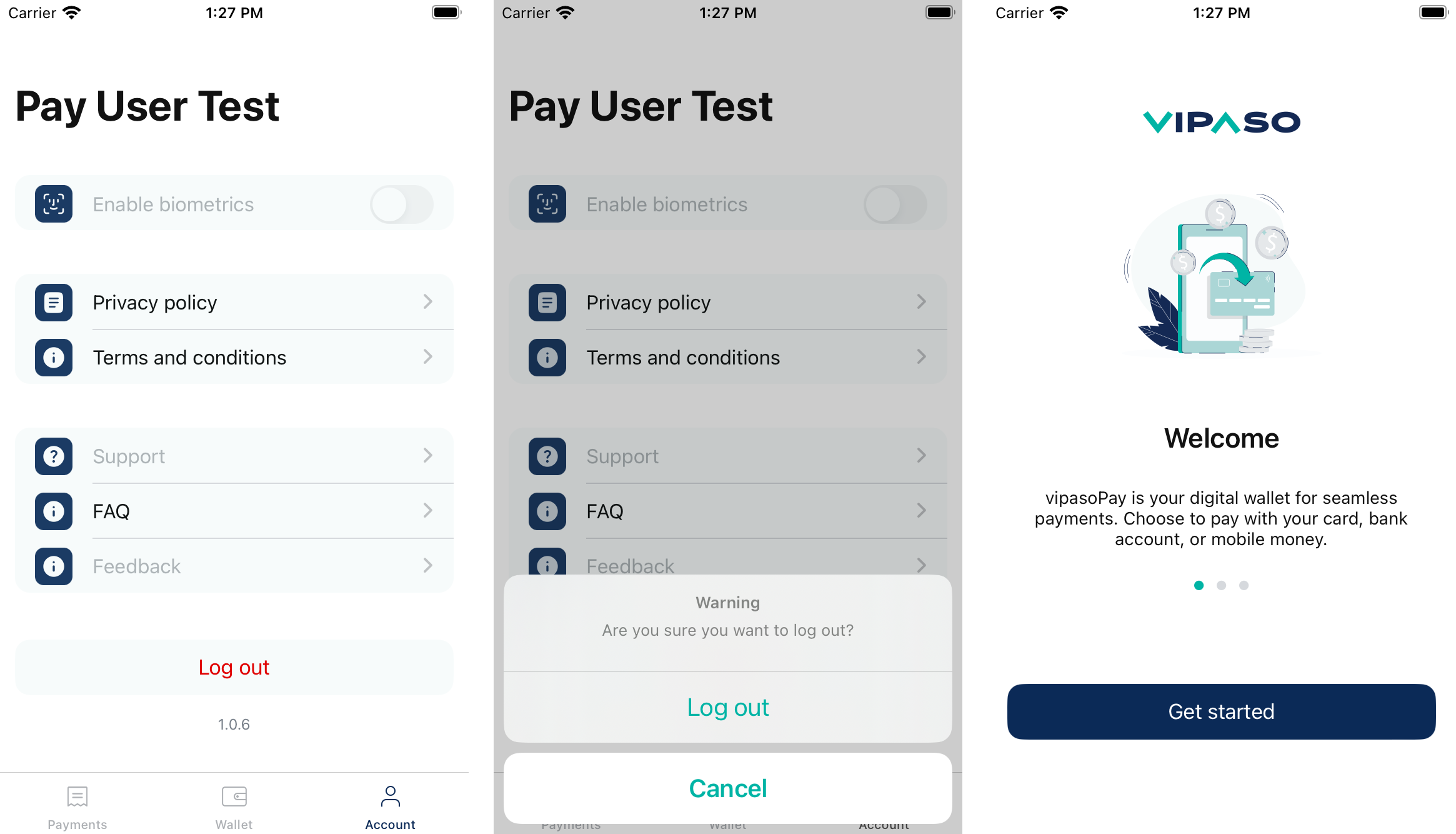
Updated 24 days ago